
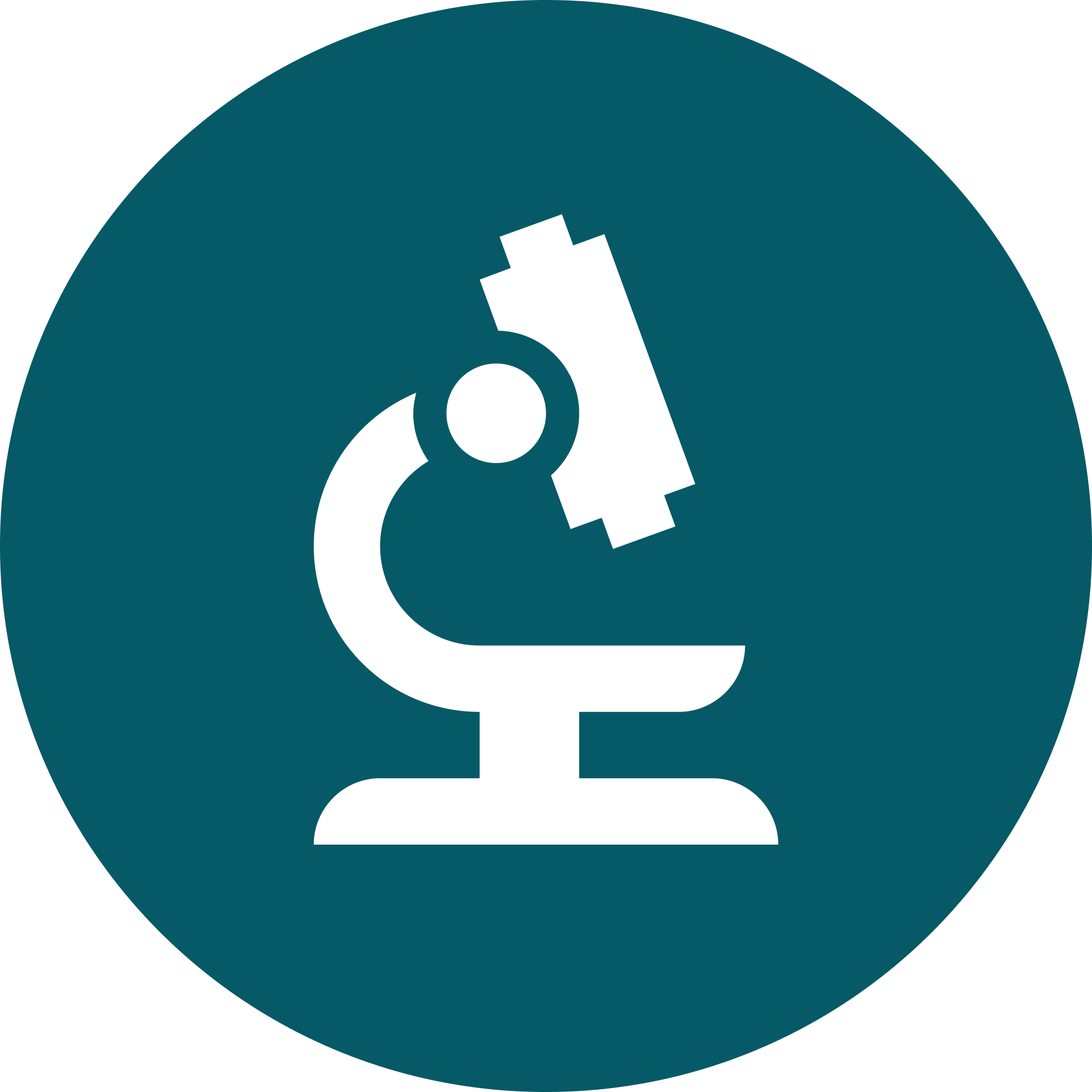
The surge of cancer since the 1900s is also explainable by the surge in our ability to detect cancer and overall understanding of it.
One big reason papers always find these links is just that they are finding correlations, which are always there, even for unrelated things. When you are looking at loads of factors in a observational study you are almost bound to find some accidental correlation. It is very hard to tell if that is just random or if there is a true cause behind it.
There are all sorts of spurious correlations if you look hard enough.
Random programming certificates are generally worthless. The course to get them might teach you a lot and be worth while, but the certificate at the end is worthless. If it is free then it does not matter too much either way, might be a good way to test yourself. But I would not rely on it to get you a job at all. For that you need other ways to prove you can do the job - typically with the ability to talk about stuff and having written some real world like application. Which a course might help you do to.